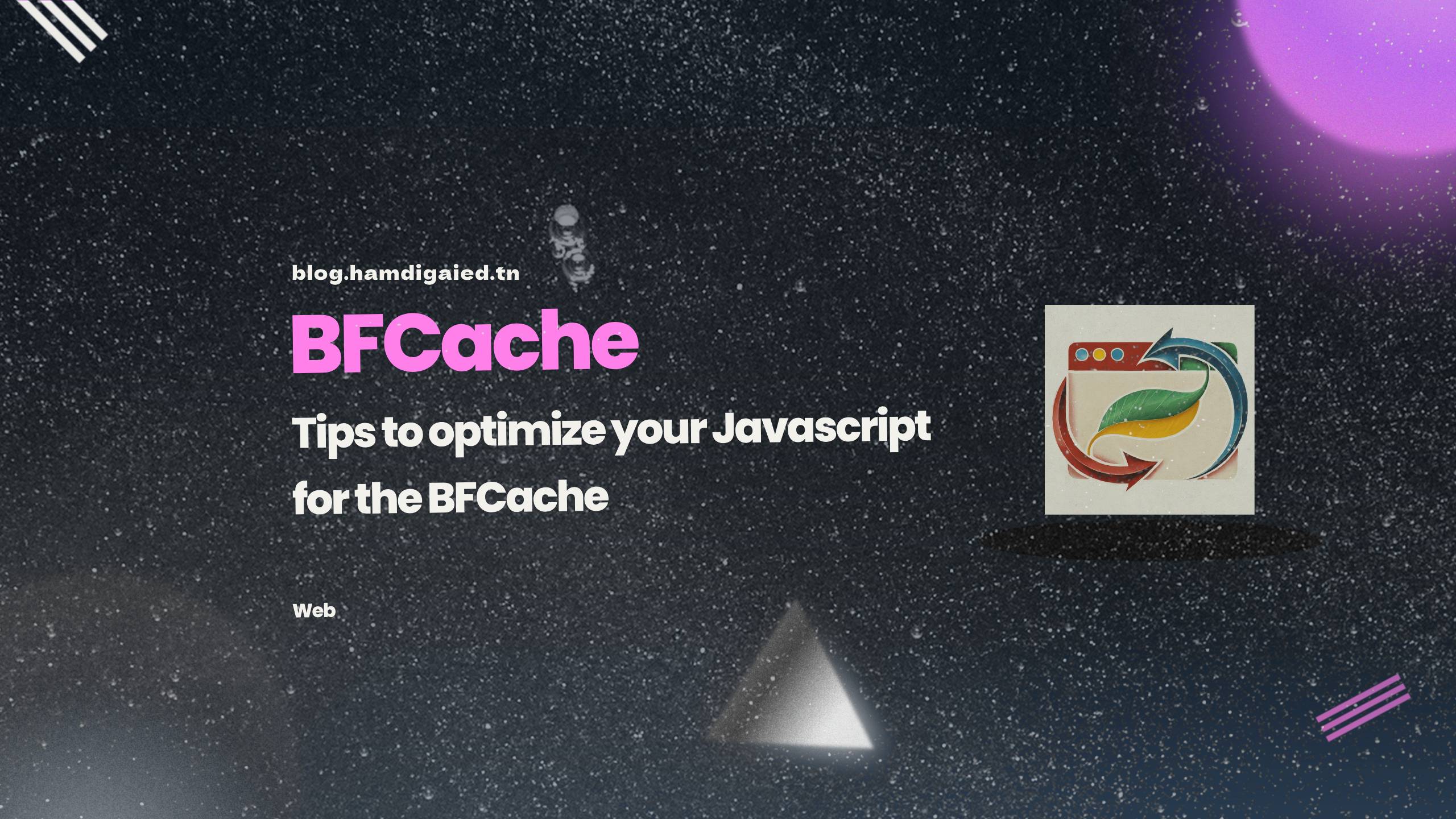
Introduction 🚀
Have you ever tried pressing the back button of the browser, and noticed how fast the previous page loads ? This is the BFCache in action.
In this article, we'll delve into what BFCache is, how it works, and strategies for writing efficient code to harness
its benefits effectively.
1. Understanding BFCache
BFCache, short for backwards/forwards cache, is a feature implemented in modern web browsers. It enables browsers to store a snapshot of a fully rendered webpage in memory when users navigate away from it, typically by clicking the back or forward buttons. When users revisit the cached page, it loads almost instantly from memory, providing a seamless and swift browsing experience.
1 in 10 navigations on desktop and 1 in 5 on mobile are either back or forward
2. How BFCache Works ?
When a user navigates away from a page, the browser captures a snapshot of the entire webpage, including its rendered HTML, CSS, JavaScript, and other assets. This snapshot is then stored in memory, allowing the browser to quickly retrieve and display the cached page when users navigate back to it.
At this point you might ask yourself: What happens to the execution of my JavaScript code ?
And that’s a very good question, because the way the BFCache works is that your entire JavaScript heap is
stored in memory, and the execution of in-progress code is paused. This means that code that’s scheduled to run with
setInterval or setTimeout will resume when the page is served from the BFCache, as if nothing happened. And the same
goes for unresolved promises.
3. Optimizing Code for BFCache
To leverage the benefits of BFCache effectively, developers can adopt several best practices :
- Minimize JavaScript Execution
Since BFCache stores the entire JavaScript heap in memory, minimizing JavaScript execution can improve performance.
Avoid long-running scripts and heavy computations that could delay page rendering. Additionally, use efficient
coding techniques and consider lazy-loading non-essential scripts to reduce initial load times.
- Listen for BFCache Lifecycle Events
Be aware of the lifecycle events associated with pages served from the BFCache. Instead of relying on traditional
page load events, listen for the pageshow
event and check the event.persisted
property to
detect when a page is restored from the cache. This allows for proper handling of stale data and dynamic content
updates.
window.addEventListener('pageshow', (event) => { if (event.persisted) { console.log('This page was restored from the bfcache.'); } else { console.log('This page was loaded normally.'); } });
- Avoid Actions that Invalidate BFCache
Certain actions, such as listening for unload events or setting cache-control
headers to
no-store
, can prevent pages from being cached in the BFCache. Avoid such actions whenever possible to
ensure that pages remain eligible for caching, thus maximizing the benefits of BFCache.
4. Testing
You can conveniently conduct BFCache tests by accessing the Chrome DevTools, navigating to the Application tab, and selecting the Back/Forward cache option. Within this section, you'll find a prominent 'test backward/forward cache button.' Clicking this button triggers Chrome to automatically navigate away from and return to your webpage, providing feedback on whether your page entered the cache or not.
If the bfcache fails, you’ll get a precise description that tells you what specifically went wrong. If it succeeds, you’ll see a green checkmark, indicating the page was successfully cached.
Conclusion ✅
If you found this article is valuable and useful, share it. 🙏♻